Testing an Express.js API with Vitest
Learn how to create super fast tests with Vitest and Supertest for your Express.js API.
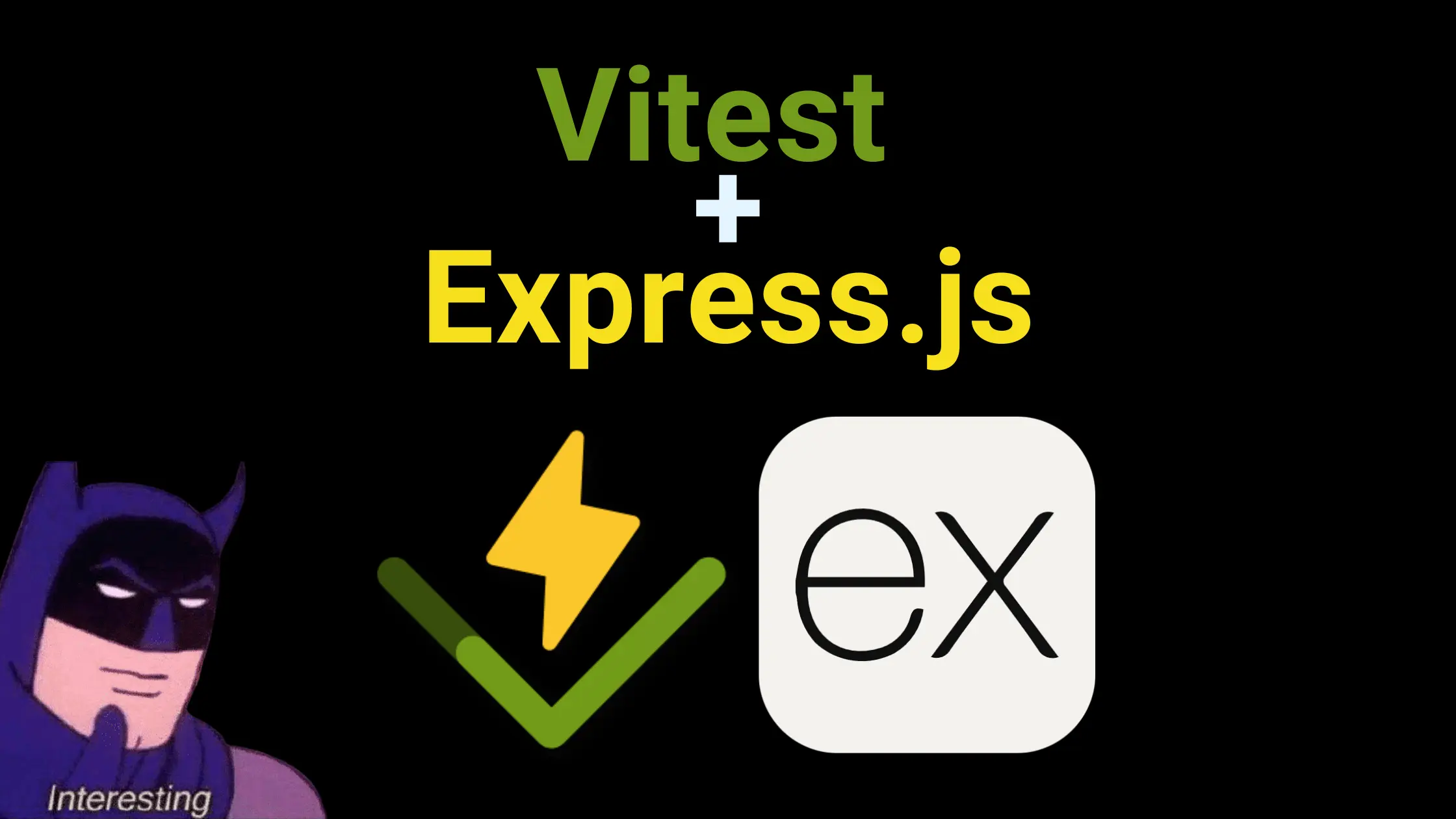
April 11, 2024
π» Vitest π» Express.js π» Supertest
Step 1: Express set up
Init the repository
Create a new directory for your project and navigate into it:
mkdir my-express-app && cd my-express-app
Then, initialize a new Node.js project:
npm init -y
Next, install the dependencies:
npm install express vitest @vitest/ui supertest dotenv
Create API files
Create a new file and add our routes so you can export them and start the server with those routes and also be able to test them:
touch root.js
Add:
import express from "express";const app = express();
app.get("/api/products", (req, res) => { res.status(200).json([ { id: 1, name: "iPhone", }, { id: 2, name: "MacBook Pro", }, ]);});
export { app };
Create the file that will initialize the server, we can run it with the βnode app.jsβ command:
touch app.js
import { app } from "./root.js";
import dotenv from "dotenv";
dotenv.config();const port = process.env.PORT || 3000;
app.listen(port, () => { console.log(`β‘ Server listening on port \x1b[33m${port}\x1b[37m`);});
Update package.json to be able to use ECMAScript modules. Your file should look like this:
{ "name": "my-express-app", "version": "1.0.0", "type": "module", "description": "", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "keywords": [], "author": "", "license": "ISC", "dependencies": { "@vitest/ui": "^1.4.0", "dotenv": "^16.4.5", "express": "^4.19.1", "supertest": "^6.3.4", "vitest": "^1.4.0" }}
Now you can test your API using the following command!
node app.js
Step 2: Vitest set up
Create Test files
Create the file that will be read by vitest and write the test:
touch index.test.js
Add:
import { test, beforeEach, afterEach } from "vitest";import { app } from "./root.js";
import request from "supertest";
let server;
beforeEach(() => { server = app.listen();});
afterEach(() => { server.close();});
test("GET /api/products", async ({ expect }) => { const response = await request(server).get("/api/products");
expect(response.statusCode).toBe(200); server.close();});
Step 3: Test your API!
To run the test execute:
npx vitest
You got it!
You can make this process easier by modifying the package.json scripts like this:
"scripts": { "test": "vitest"},