Learn to implement scheduled email sending with Spring Boot and Resend
In a simple way, send emails through a REST API with Spring Boot and Resend.
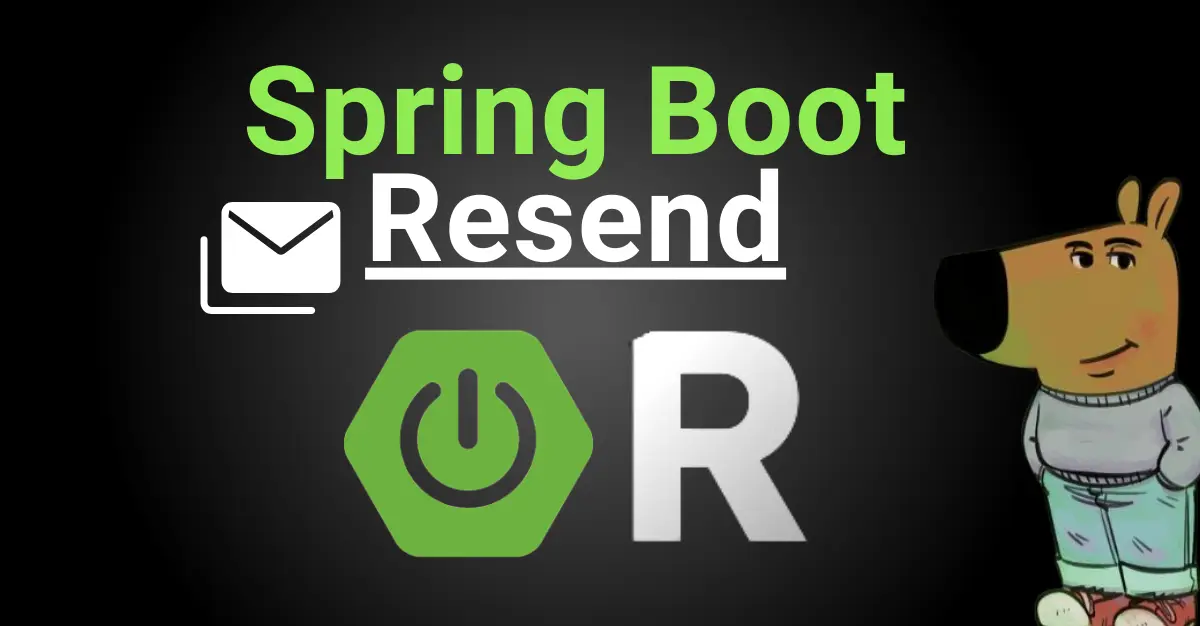
December 23, 2024
Table of Contents
- Introduction
- Step 1: Creating the Spring Boot project
- Step 2: Implementing Resend as a dependency and configuration
- Step 3: Email service
- Step 4: Scheduler
- Step 5: Conclusion
- References
Introduction
Resend is a technology that allows you to integrate email sending in a simple and worry-free manner regarding infrastructure. In this tutorial, you will learn to implement a scheduled task in Spring Boot to send emails easily.
Step 1: Creating the Spring Boot project
To start, we need to create a Spring Boot project. For this, you can use Spring Initializr. Make sure to select the following dependencies:
- Spring Web
- Spring Boot DevTools
After that, download and unzip it in your working directory. Next, open your project in your favorite IDE or text editor; I will be using Visual Studio Code.
Step 2: Implementing Resend as a dependency and configuration
Open the pom.xml
file and add the following dependency:
<dependency> <groupId>com.resend</groupId> <artifactId>resend-java</artifactId> <version>3.1.0</version></dependency>
It should look like this:
<?xml version="1.0" encoding="UTF-8"?><project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>3.4.1</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.example</groupId> <artifactId>demo</artifactId> <version>0.0.1-SNAPSHOT</version> <name>demo</name> <description>Demo project for Spring Boot</description> <url/> <licenses> <license/> </licenses> <developers> <developer/> </developers> <scm> <connection/> <developerConnection/> <tag/> <url/> </scm> <properties> <java.version>23</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> <optional>true</optional> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>com.resend</groupId> <artifactId>resend-java</artifactId> <version>3.1.0</version> </dependency> </dependencies>
<build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build></project>
Step 3: Email service
We need to create a service that sends emails. To do this, create a class called EmailService
in the com.example.demo.service
package and add the following code:
package com.example.demo.service;
import com.resend.*;import com.resend.core.exception.ResendException;import com.resend.services.emails.model.CreateEmailOptions;
import org.springframework.beans.factory.annotation.Value;import org.springframework.stereotype.Service;
@Servicepublic class EmailService { @Value("${resend.token}") private String emailToken;
@Value("${resend.email.to}") private String emailTo;
public void sendEmail() { Resend resend = new Resend(emailToken);
try { CreateEmailOptions params = CreateEmailOptions.builder() .to(emailTo) .subject("it works!") .html("<h1>Hi there!</h1><p>Here's your email from Resend</p> <p>SUSCRIBE TO ERICKDEVV</p>") .build(); resend.emails().send(params); } catch (ResendException e) { e.printStackTrace(); } }}
As you can see, we have created a sendEmail
method that sends an email using Resend. For this, we need the Resend token, which is stored in the application.properties
file. Next, add the following properties in the application.properties
file:
resend.token=<your_resend_token>resend.email.to=<the_email_to_send>
You can obtain the Resend token on the Resend page. Register and get your token in the API Keys/Create API Key section, click on Create API Key.
Then, fill in the fields and click on Add
.
Finally, copy your token.
Replace <your_resend_token>
and <the_email_to_send>
with your Resend token and the email address you want to send the email to, respectively.
Now, create a META-INF
folder in resources and within it, the additional-spring-configuration-metadata.json
file with the following content:
{ "properties": [ { "name": "resend.email.to", "type": "java.lang.String", "description": "The email address to send the email to" }, { "name": "resend.token", "type": "java.lang.String", "description": "The Resend token" } ]}
The above is necessary for Spring Boot to recognize the properties we have defined in the application.properties
file.
Step 4: Scheduler Task
Now itโs time to create the scheduled task that runs at regular intervals. To do this, create a class called EmailScheduler
in the com.example.demo.scheduler
package and add the following code:
package com.example.demo.scheduler;
import org.springframework.beans.factory.annotation.Autowired;import org.springframework.scheduling.annotation.Scheduled;import org.springframework.stereotype.Component;
import com.example.demo.service.EmailService;
@Componentpublic class EmailScheduler {
@Autowired private EmailService emailService;
@Scheduled(cron = "0 */5 * * * *", zone = "America/Mexico_City") public void sendEmail() { try { emailService.sendEmail(); System.out.println("Email sent successfully"); } catch (Exception e) { System.out.println("Error sending email: " + e.getMessage()); } }}
We have created a sendEmail
method that runs every 5 minutes. You can customize the time interval according to your needs. Additionally, we have injected the EmailService
dependency to send emails and defined the time zone as Mexico City (you can change it according to your location).
We need to add the @EnableScheduling
annotation in a configuration class to enable scheduling in Spring Boot. To do this, create a class called SchedulingConfig
in the com.example.demo.config
package and add the following code:
package com.example.demo.config;
import org.springframework.context.annotation.Configuration;import org.springframework.scheduling.annotation.EnableScheduling;
@Configuration@EnableSchedulingpublic class SchedulingConfig {}
Now, run your Spring Boot application, and you will see that an email is sent every 5 minutes to the specified email address.
Step 5: Conclusion
Resend is a tool that integrates very easily with Spring Boot applications, is really powerful, and does not require any additional infrastructure. Personally, I have enjoyed working with Resend, and I hope you do too.
Happy coding!